เกริ่นนำก่อน ผมได้โจทย์มาจากตอนสัมภาษณ์งาน มีทั้งที่ให้ผู้สัมภาษณ์ช่วย และทำได้เอง มาดูกันดีกว่าาาาา
1. หาตัวเลขที่ผลรวมใน array ที่เท่ากับ goal
let InputAry = [2, 5, 22, 10, 15, 11];
const Goal = 38;
// output -> [5,22,11], [2,10,15,11]
let InputAry = [2, 5, 22, 10, 15, 11];
const Goal = 38;
function SUM(member) {
return member.reduce((total, num) => total + num);
}
// root = ราก
// numAry = node ของ root -> ซึ่งจะมากลายเป็น root ในบรรทัดที่ 42
// goal = เป้าหมายที่ต้องการ
// member = จำนวนสมาชิกที่ต้องเป็นเลขจาก InputAry
// หลักการก็คือ ใช้ recursive tree
// โดยส่ง myGoal(root + numAry[i], numAryExcludRoot, goal, cloneMember);
// root + numAry[i] จะเท่ากับ root ใหม่
// numAryExcludeRoot = numAry แต่ excludeRoot -> ซึ่งสุดท้ายแล้วมันจะไปสิ้นสุดที่ [] เป็นค่าว่าง
// cloneMember ก็ member นะแหละ แต่ต้องโคลนเพราะ javascript มัน pass by reference (บ้ามากกก ผมติดตรงนี้นานมาก)
function myGoal(root, numAry, goal, member) {
let newMember = root - SUM(member);
if (newMember < 0) {
// console.log(root, newMember, member);
member = [root];
}
member.push(newMember);
if (numAry.length === 0) {
return { isGoal: false, m: member };
}
if (root === goal) {
console.log('success', member);
return { isGoal: true, m: member };
// return { isGoal: true, m: member.concat(root - SUM(member)), numAry };
}
if (root > goal) {
return { isGoal: false, m: member };
}
// Logic
for (let i = 0; i < numAry.length; i++) {
let numAryExcludeRoot = JSON.parse(JSON.stringify(numAry));
let cloneMember = JSON.parse(JSON.stringify(member));
numAryExcludeRoot.splice(i, 1);
const result = myGoal(root + numAry[i], numAryExcludeRoot, goal, cloneMember);
if ((result) && (result.isGoal)) {
// return result;
}
}
}
const result = myGoal(0, InputAry, Goal, [0]);
if (result) {
console.log('result', result);
}
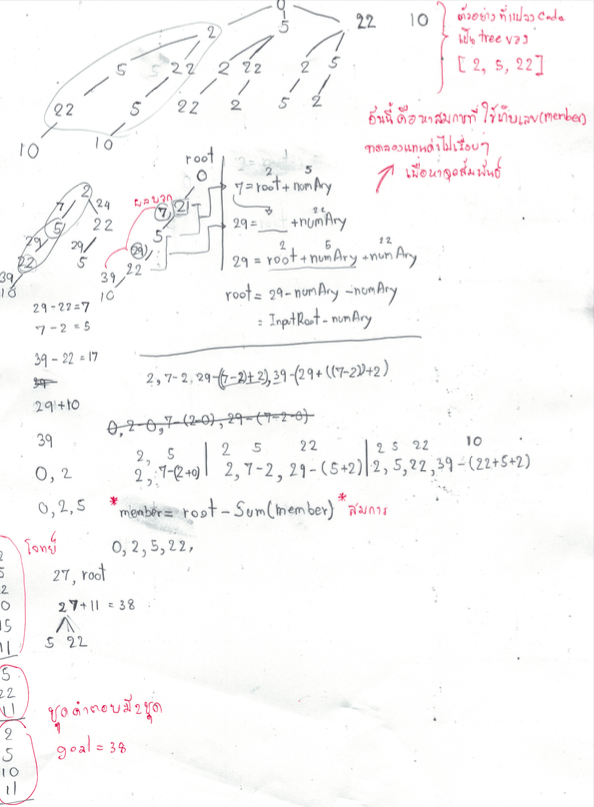
2. ทำการ sort array โดยไม่เปิด google ไม่ใช้ lib
//Sorting array
let InputAry = [1, 3, 7, 5, 11, 9];
// output -> [1, 3, 5, 7, 9, 11]
//Sorting array
let InputAry = [1, 3, 7, 5, 11, 9];
// output -> [1, 3, 5, 7, 9, 11]
// หลักการคือ ใช้ 2 array
// เลยมี 2 for เพื่อวนเทียบกัน
// array i [1, 3, 7, 5, 11, 9]
// array j [1, 3, 7, 5, 11, 9]
// ตัวอย่างเช่นจังหวะ i7 เทียบ j5 ก็จะทำการสลับ
// โดยฝากไว้ที่ tmp แล้วแลกค่า ซึ่ง array i ก็จะได้หน้าตาใหม่
// เป็น array i [1, 3, 5, 7, 11, 9] แล้วก็วนไปเรื่อยๆครับ
function sort(input) {
if (input.length === 0) {
return;
}
for (let i = 0; i < input.length; i++) {
for (let j = 0; j < (input.length - i); j++) {
// Logic
if (input[i] > input[i + j]) {
const tmp = input[i];
input[i] = input[i + j];
input[i + j] = tmp;
}
}
console.log('i', input);
}
}
function main() {
sort(InputAry);
}
main();
จบแล้วววววว จริงๆแล้ว ผมคิดว่าการทดสอบด้วยโจทย์ มันก็วัดความชำนาญในการโค้ดและโลจิกได้ แต่ในโลกการทำงานจริง ผมแทบไม่ได้เขียนโลจิกพวกนี้เลย เพราะหลายๆภาษาก็มีทั้ง standard lib, 3party lib ให้ใช้
ซึ่งปัญหาในการทำงาน จะเน้นไปการ Good problem-solving skills มากกว่าครับ